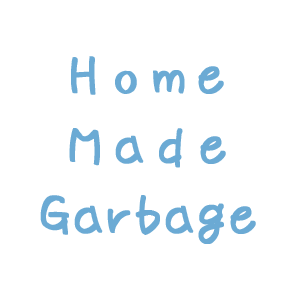
I added a motor and a microphone, and a wiring diagram is generated as shown in the following figure.
https://storage.circuito.io/index.html?solutionId=58ece9cc4e95b700128641cb
Wired according to the figure.

Parts
- Microcomputer Arduino uno
- Elecelet mike module
- Pump motor
- Power nMOS
- Resistor 10kohm
Arduino IDE program
I modified the program generated by the circuito.io as follows.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
// Include Libraries #include "Arduino.h" #include "Mic.h" #include "Pump.h" // Pin Definitions #define MIC_PIN_SIG A3 #define WATERPUMP_PIN_COIL1 5 // Global variables and defines // Constructors Mic mic(MIC_PIN_SIG); Pump waterpump(WATERPUMP_PIN_COIL1); /* This code sets up the essentials for your circuit to work. It runs first every time your circuit is powered with electricity. */ void setup() { // Setup Serial which is useful for debugging // Use the Serial Monitor to view printed messages Serial.begin(9600); } /* This code is the main logic of your circuit. It defines the interaction between the components you selected. After setup, it runs over and over again, in an eternal loop. */ void loop() { //Mic int micVal = abs(mic.read() -500); Serial.print(F("Val: ")); Serial.println(micVal); //SubWaterPump if(micVal > 400){ waterpump.on(); // turns on }else{ waterpump.off();// turns off } } |
Operation
Place a microphone in a broken balloon and attach a balloon to the motor. Blowing a broken balloon inflate the other balloon.