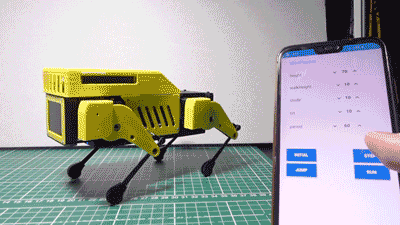
ロボット犬『Mini Pupperミニぷぱ』Pythonでモーション記述
前回はミニぷぱ正規の構成でラズパイ4にRaspi OSとNode-REDを導入して動作を確認いたしました。
ここでは、動作モーションを増やしてのコントロールを検討します。
目次
モーション制御検討
Python
前回はNode-REDで動作を仕込みましたが、複数のモーションになると煩雑になるためPythonを採用することにしました。
サーボドライバ PCA9685用のPythonライブラリは以下を使用しました。
https://github.com/adafruit/Adafruit_Python_PCA9685
ちなみにこのライブラリでも専用基板のサーボナンバー 1~12がPCA9685ノードの15~4に対応していました。基板上そういった接続なっているのかもしれません。
Node-RED Dashboard
ミニぷぱコントロールUIにはNode-RED-Dashboardを使用することにしました。
https://flows.nodered.org/node/node-red-dashboard
これによってブラウザ上のボタンなどで制御可能となります。
動作
Node-RED-Dashboardのボタンで足の高さを指定しています。
ブラウザで動くのでスマホでもコントロールできます。
逆運動学によるモーションはpythonで書いて
UIはnode-red-dashboardにする
そうすればスマホでもコントロールできる#MiniPupper #ミニぷぱ #nodeRED #python pic.twitter.com/cFF8FWf7G0— HomeMadeGarbage (@H0meMadeGarbage) February 17, 2022
ミニぷぱモーション制御
Dashboard
Node-RED Dashboardのnumericノードで足の高さや上げ幅、歩幅などを指定します。
buttonノードで各種モーションを起動します。
モーションは初期姿勢、足踏み、ジャンプ、ランニングマンを用意しました。
Node-RED
Node-REDの構成は以下のとおり
動作指定ボタンを受けて各モーションのPythonコードを起動しています。
Python起動時にnumericノードで指定したパラメータをmsg.payloadで引数として渡しています。
Pythonコード
足踏み動作は以下のような感じで
参考
動作
足踏みやジャンプを仕込んで
いよいよ前後左右への歩行
dashboardにはジョイスティックがなく
ボタンやスライダじゃきついなぁと思った時
Blynkはラズパイもいけたことに気づく。。ラズパイ4に対応してるかは明日のおかずだな#MiniPupper #ミニぷぱ #nodeRED #python pic.twitter.com/ybpuc5jLUj
— HomeMadeGarbage (@H0meMadeGarbage) February 17, 2022
前後左右への歩行を仕込もうと思ったのですがdashboardにはジョイスティックがなくボタンやスライダじゃきついなぁと思った時に
Blynkはラズパイもいけたことに気づきました。。。
次はBlynkでの動作検証します。
おわりに
ここではNode-RED DashboardとPythonを用いてモーションコントロールを楽しみました。
しかしNode-RED DashboardのUIではリアルタイムラジコンを制御するには少し物足りない印象でした。
Blynkがラズパイ対応していたことに今さら気づいたので次回検証進めます!
追記 (ディスプレイ表示)
どうもラズパイ4はBlynkに対応されてないようなので、ラズパイを用いた本構成の検討はコレで一旦休止しようと思います。
最後にせっかくなのでディスプレイ表示だけ確認しました。
ディスプレイには320×240ピクセルのST7789が使用されていることが以下で解ります。
SPIで制御されておりピン配もPythonコードから読み取ることができます。
https://github.com/mangdangroboticsclub/QuadrupedRobot/tree/MiniPupper_V2/Mangdang/LCD
ST7789制御
以下を参考にST7789用Python環境を構築しました。
またRaspiコンフィグでSPIを有効にします。
Pythonコード
上記を参考にして320×240のJPEG画像を表示するPythonコードをこしらえました。
動作
ディスプレイ表示できるように なたよ#MiniPupper #ミニぷぱ #ST7789 #python #ペットボトル pic.twitter.com/8aJwefMM3A
— HomeMadeGarbage (@H0meMadeGarbage) February 18, 2022
表示できました!
思えばラズパイにLCDつないでSPIで制御したの初めてかも
勉強になりました!