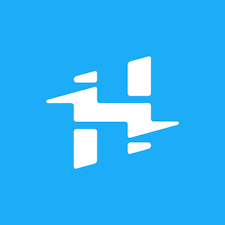
RedBear
hackster.io への投稿方法
hacksterは自作ハードウェア作品の紹介記事やデバイスやツールの使用方法を投稿できるコミュニケーションサイトです。 [bc url ="https://www.hackster.io/"] FacebookやTwitter等のSNSアカウントでもアカウント登録可能です。 様々な電子作品やツ...
由运动传感器和华踏板控制的 ble 车
我做了一辆由运动传感器和 wah 踏板控制的 ble 汽车。 配置 部分 Redbearlab BLE Nano [amazonjs asin="B01G5EZTVE" locale="JP" title="RedBear DUO – Wi-Fi + BLE IoT Board"]...
ワウペダルと加速度センサでBLE制御ラジコン!
以前スマホでコントロールするラジコンを作ったのですが。。。 https://homemadegarbage.com/blynk_car01 やっぱり物理コントローラで動かしてみたくなりハンドルに加速度センサ、アクセルに大昔に買ったワウペダルを使ってコントローラつくりました♪コントローラと車の通信...
BLE Car Controlled by Motion Sensor and Wah Pedal
I made a BLE car controlled by motion sensor and wah pedal. Configuration Parts Redbearlab BLE Nano [amazonjs asin="B01G5EZTVE" locale="JP" ...
Blynkでダイソー版プラレールコントロール!
いつのまにかBlynkがBLE対応してた!(まだβ版のようだが) ということで早速以前作ったBLEトレインをBlynkで動かしてみましたので記載します。 Blynkに関する過去記事はこちら https://homemadegarbage.com/esp-wroom-blynk BL...
BLE Nano – Redbear Duo間通信でモータ&LED制御 w/ Android -球体POV製作への道 その6-
前回、Redbear DuoでLEDテープ(Dotstar)を制御できるようにしました。んで今回は ステップ4:スマホ – Redbear Duo でLED色・回転速度WiFi制御かつ Redbear Duo – BLE Nanoでモーター速度制御&測定 です。いやー手こず...
Redbear DuoでDotstarを制御 -球体POV製作への道 その5-
前回 BLE通信使用してRedbear Duoでモーター供給電圧を指定してBLE Nanoでモーターを回転&回転スピードDuoへ送信が確認しました。んで今回は ステップ3:Redbear DuoでLEDテープ(Dotstar)を制御 です。新しいコアモジュールでこの手のLED...
BLE Nano – Redbear Duo間通信でモータ制御 -球体POV製作への道 その4-
前回 BLE Nanoとスマホでモーターの回転スピード制御するところまで出来ましたので今回は ステップ2:BLE Nano (Peripheral) - Redbear Duo (Central) のBLE通信でDuoで モーター供給電圧を指定してBLE Nanoから回転スピ...
BLE NanoでDCモータ制御 w/ Android -球体POV製作への道 その3-
さて随分あいだがあいてしまいましたがPOV再開です。 https://homemadegarbage.com/pov-sphere01 前回ステッピングモータを使用してみたのですが全然スピード出なかったので方針変更して DCモーターのスピードをBLE Nano介してスマホでコントロール ...
BLE Nanoでステッピングモータ制御 -球体POV製作への道 その2-
前回 考えた仕様の検証ステップ1ということで BLE Nano(https://www.switch-science.com/catalog/2403/)でステッピングモータ制御 の確認をしたいと思います。 BLE Nanoからパルス出してモータドライバをコントロールします。せっ...
球体POV製作への道 その1
以下のような球体のPOV(Persistent Of Vision)装置を作りたくこちらで 進捗など記載できればとおもいペンをとります。 POV(Persistent Of Vision)とはLEDなどを高速に動かして残像で映像を表示するものです。 システム(あくまで理想) ...
BLE制御ダイソー版 プラレール製作工程
先日Maker Faire Bay Area 2016でも展示していただいたBLEトレインの製作工程を紹介します! [bc url ="https://homemadegarbage.com/mfba16"] BLE電車概要 1両目に左右に歩行転換するためのサーボモータとバッテリー...
Maker Faire Bay Area 2016 RedBear社ブースに協力させて頂きました
スマホで動く電車のおもちゃを作って動画やブログをアップしたのですが [bc url ="https://homemadegarbage.com/ble-plarail"] なんとこの電車に利用したBLE Nanoを製造・販売しているRed Bear Company様からMaker Faire B...
WiFi MicroとBlynkで室温記録
してみました! システム概要 DS3231ボードをI2CでWiFi Microにつないで温度情報をBlynkアプリでプロットします。 WiFi Microの詳細についでは以下も参照ください。 [bc url = "https://homemadegarbage.com/...
RedBearLab WiFi Micro の設定方法 for Windows
WiFi Microとは RedBear社のTI製WiFiコアCC3200搭載の小型マイコンです。 上に刺さってるのがWiFi Microで下の基板がUSB書き込み用ボードのMK20 購入先 [amazonjs asin="B018M1F1J8" locale="JP" ...